mirror of
https://github.com/RetroDECK/Duckstation.git
synced 2024-10-23 23:55:42 +00:00
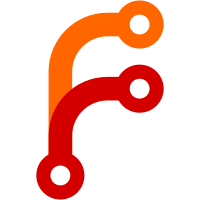
* CPU/Recompiler: Use rel32 call where possible for no-args * JitCodeBuffer: Support using preallocated buffer * CPU/Recompiler/AArch64: Use bl instead of blr for short branches * CPU/CodeCache: Allocate recompiler buffer in program space This means we don't need 64-bit moves for every call out of the recompiler. * GTE: Don't store as u16 and load as u32 * CPU/Recompiler: Add methods to emit global load/stores * GTE: Convert class to namespace * CPU/Recompiler: Call GTE functions directly * Settings: Turn into a global variable * GPU: Replace local pointers with global * InterruptController: Turn into a global pointer * System: Replace local pointers with global * Timers: Turn into a global instance * DMA: Turn into a global instance * SPU: Turn into a global instance * CDROM: Turn into a global instance * MDEC: Turn into a global instance * Pad: Turn into a global instance * SIO: Turn into a global instance * CDROM: Move audio FIFO to the heap * CPU/Recompiler: Drop ASMFunctions No longer needed since we have code in the same 4GB window. * CPUCodeCache: Turn class into namespace * Bus: Local pointer -> global pointers * CPU: Turn class into namespace * Bus: Turn into namespace * GTE: Store registers in CPU state struct Allows relative addressing on ARM. * CPU/Recompiler: Align code storage to page size * CPU/Recompiler: Fix relative branches on A64 * HostInterface: Local references to global * System: Turn into a namespace, move events out * Add guard pages * Android: Fix build
154 lines
3.2 KiB
C++
154 lines
3.2 KiB
C++
#pragma once
|
|
#include "controller.h"
|
|
#include <array>
|
|
#include <memory>
|
|
#include <optional>
|
|
#include <string_view>
|
|
|
|
class AnalogController final : public Controller
|
|
{
|
|
public:
|
|
enum class Axis : u8
|
|
{
|
|
LeftX,
|
|
LeftY,
|
|
RightX,
|
|
RightY,
|
|
Count
|
|
};
|
|
|
|
enum class Button : u8
|
|
{
|
|
Select = 0,
|
|
L3 = 1,
|
|
R3 = 2,
|
|
Start = 3,
|
|
Up = 4,
|
|
Right = 5,
|
|
Down = 6,
|
|
Left = 7,
|
|
L2 = 8,
|
|
R2 = 9,
|
|
L1 = 10,
|
|
R1 = 11,
|
|
Triangle = 12,
|
|
Circle = 13,
|
|
Cross = 14,
|
|
Square = 15,
|
|
Analog = 16,
|
|
Count
|
|
};
|
|
|
|
static constexpr u8 NUM_MOTORS = 2;
|
|
|
|
AnalogController(u32 index);
|
|
~AnalogController() override;
|
|
|
|
static std::unique_ptr<AnalogController> Create(u32 index);
|
|
static std::optional<s32> StaticGetAxisCodeByName(std::string_view axis_name);
|
|
static std::optional<s32> StaticGetButtonCodeByName(std::string_view button_name);
|
|
static AxisList StaticGetAxisNames();
|
|
static ButtonList StaticGetButtonNames();
|
|
static u32 StaticGetVibrationMotorCount();
|
|
static SettingList StaticGetSettings();
|
|
|
|
ControllerType GetType() const override;
|
|
std::optional<s32> GetAxisCodeByName(std::string_view axis_name) const override;
|
|
std::optional<s32> GetButtonCodeByName(std::string_view button_name) const override;
|
|
|
|
void Reset() override;
|
|
bool DoState(StateWrapper& sw) override;
|
|
|
|
void SetAxisState(s32 axis_code, float value) override;
|
|
void SetButtonState(s32 button_code, bool pressed) override;
|
|
|
|
void ResetTransferState() override;
|
|
bool Transfer(const u8 data_in, u8* data_out) override;
|
|
|
|
void SetAxisState(Axis axis, u8 value);
|
|
void SetButtonState(Button button, bool pressed);
|
|
|
|
u32 GetVibrationMotorCount() const override;
|
|
float GetVibrationMotorStrength(u32 motor) override;
|
|
|
|
void LoadSettings(const char* section) override;
|
|
|
|
private:
|
|
using MotorState = std::array<u8, NUM_MOTORS>;
|
|
|
|
enum class State : u8
|
|
{
|
|
Idle,
|
|
GetStateIDMSB,
|
|
GetStateButtonsLSB,
|
|
GetStateButtonsMSB,
|
|
GetStateRightAxisX,
|
|
GetStateRightAxisY,
|
|
GetStateLeftAxisX,
|
|
GetStateLeftAxisY,
|
|
ConfigModeIDMSB,
|
|
ConfigModeSetMode,
|
|
SetAnalogModeIDMSB,
|
|
SetAnalogModeVal,
|
|
SetAnalogModeSel,
|
|
GetAnalogModeIDMSB,
|
|
GetAnalogMode1,
|
|
GetAnalogMode2,
|
|
GetAnalogMode3,
|
|
GetAnalogMode4,
|
|
GetAnalogMode5,
|
|
GetAnalogMode6,
|
|
UnlockRumbleIDMSB,
|
|
Command46IDMSB,
|
|
Command461,
|
|
Command462,
|
|
Command463,
|
|
Command464,
|
|
Command465,
|
|
Command466,
|
|
Command47IDMSB,
|
|
Command471,
|
|
Command472,
|
|
Command473,
|
|
Command474,
|
|
Command475,
|
|
Command476,
|
|
Command4CIDMSB,
|
|
Command4CMode,
|
|
Command4C1,
|
|
Command4C2,
|
|
Command4C3,
|
|
Command4C4,
|
|
Command4C5,
|
|
Pad6Bytes,
|
|
Pad5Bytes,
|
|
Pad4Bytes,
|
|
Pad3Bytes,
|
|
Pad2Bytes,
|
|
Pad1Byte,
|
|
};
|
|
|
|
u16 GetID() const;
|
|
void SetAnalogMode(bool enabled);
|
|
void SetMotorState(u8 motor, u8 value);
|
|
|
|
u32 m_index;
|
|
|
|
bool m_auto_enable_analog = false;
|
|
|
|
bool m_analog_mode = false;
|
|
bool m_analog_locked = false;
|
|
bool m_rumble_unlocked = false;
|
|
bool m_configuration_mode = false;
|
|
u8 m_command_param = 0;
|
|
|
|
std::array<u8, static_cast<u8>(Axis::Count)> m_axis_state{};
|
|
|
|
// buttons are active low
|
|
u16 m_button_state = UINT16_C(0xFFFF);
|
|
|
|
MotorState m_motor_state{};
|
|
|
|
State m_state = State::Idle;
|
|
};
|