mirror of
https://github.com/RetroDECK/Duckstation.git
synced 2024-10-23 15:45:42 +00:00
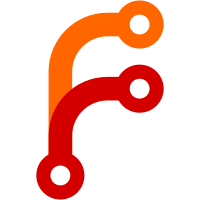
* CPU/Recompiler: Use rel32 call where possible for no-args * JitCodeBuffer: Support using preallocated buffer * CPU/Recompiler/AArch64: Use bl instead of blr for short branches * CPU/CodeCache: Allocate recompiler buffer in program space This means we don't need 64-bit moves for every call out of the recompiler. * GTE: Don't store as u16 and load as u32 * CPU/Recompiler: Add methods to emit global load/stores * GTE: Convert class to namespace * CPU/Recompiler: Call GTE functions directly * Settings: Turn into a global variable * GPU: Replace local pointers with global * InterruptController: Turn into a global pointer * System: Replace local pointers with global * Timers: Turn into a global instance * DMA: Turn into a global instance * SPU: Turn into a global instance * CDROM: Turn into a global instance * MDEC: Turn into a global instance * Pad: Turn into a global instance * SIO: Turn into a global instance * CDROM: Move audio FIFO to the heap * CPU/Recompiler: Drop ASMFunctions No longer needed since we have code in the same 4GB window. * CPUCodeCache: Turn class into namespace * Bus: Local pointer -> global pointers * CPU: Turn class into namespace * Bus: Turn into namespace * GTE: Store registers in CPU state struct Allows relative addressing on ARM. * CPU/Recompiler: Align code storage to page size * CPU/Recompiler: Fix relative branches on A64 * HostInterface: Local references to global * System: Turn into a namespace, move events out * Add guard pages * Android: Fix build
87 lines
2.4 KiB
C++
87 lines
2.4 KiB
C++
#pragma once
|
|
#include "common_host_interface.h"
|
|
#include "core/types.h"
|
|
#include <array>
|
|
#include <functional>
|
|
#include <map>
|
|
#include <mutex>
|
|
|
|
class HostInterface;
|
|
class Controller;
|
|
|
|
class ControllerInterface
|
|
{
|
|
public:
|
|
enum : int
|
|
{
|
|
MAX_NUM_AXISES = 7,
|
|
MAX_NUM_BUTTONS = 15
|
|
};
|
|
|
|
using AxisCallback = CommonHostInterface::InputAxisHandler;
|
|
using ButtonCallback = CommonHostInterface::InputButtonHandler;
|
|
|
|
ControllerInterface();
|
|
virtual ~ControllerInterface();
|
|
|
|
virtual bool Initialize(CommonHostInterface* host_interface);
|
|
virtual void Shutdown();
|
|
|
|
// Removes all bindings. Call before setting new bindings.
|
|
virtual void ClearBindings() = 0;
|
|
|
|
// Binding to events. If a binding for this axis/button already exists, returns false.
|
|
virtual bool BindControllerAxis(int controller_index, int axis_number, AxisCallback callback) = 0;
|
|
virtual bool BindControllerButton(int controller_index, int button_number, ButtonCallback callback) = 0;
|
|
virtual bool BindControllerAxisToButton(int controller_index, int axis_number, bool direction,
|
|
ButtonCallback callback) = 0;
|
|
|
|
virtual void PollEvents() = 0;
|
|
|
|
// Changing rumble strength.
|
|
virtual u32 GetControllerRumbleMotorCount(int controller_index) = 0;
|
|
virtual void SetControllerRumbleStrength(int controller_index, const float* strengths, u32 num_motors) = 0;
|
|
|
|
// Set scaling that will be applied on axis-to-axis mappings
|
|
virtual bool SetControllerAxisScale(int controller_index, float scale) = 0;
|
|
|
|
// Set deadzone that will be applied on axis-to-button mappings
|
|
virtual bool SetControllerDeadzone(int controller_index, float size) = 0;
|
|
|
|
// Input monitoring for external access.
|
|
struct Hook
|
|
{
|
|
enum class Type
|
|
{
|
|
Axis,
|
|
Button
|
|
};
|
|
|
|
enum class CallbackResult
|
|
{
|
|
StopMonitoring,
|
|
ContinueMonitoring
|
|
};
|
|
|
|
using Callback = std::function<CallbackResult(const Hook& ei)>;
|
|
|
|
Type type;
|
|
int controller_index;
|
|
int button_or_axis_number;
|
|
float value; // 0/1 for buttons, -1..1 for axises
|
|
};
|
|
void SetHook(Hook::Callback callback);
|
|
void ClearHook();
|
|
|
|
protected:
|
|
bool DoEventHook(Hook::Type type, int controller_index, int button_or_axis_number, float value);
|
|
|
|
void OnControllerConnected(int host_id);
|
|
void OnControllerDisconnected(int host_id);
|
|
|
|
CommonHostInterface* m_host_interface = nullptr;
|
|
|
|
std::mutex m_event_intercept_mutex;
|
|
Hook::Callback m_event_intercept_callback;
|
|
};
|