mirror of
https://github.com/RetroDECK/ES-DE.git
synced 2024-11-23 22:55:39 +00:00
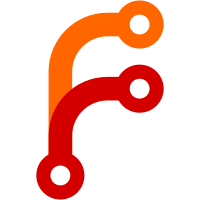
SDL_mixer is not in the standard SDL distribution. The mixing is now done using regular SDL_Audio functions. AudioManager is converted to a singleton and std::shared_ptrs are used for all Sound objects. Note that for GCC "-std=c++11" might need to be added to the CMAKE_CXX_FLAGS.
37 lines
559 B
C++
37 lines
559 B
C++
#ifndef _SOUND_H_
|
|
#define _SOUND_H_
|
|
|
|
#include <string>
|
|
#include "SDL_audio.h"
|
|
|
|
|
|
class Sound
|
|
{
|
|
std::string mPath;
|
|
SDL_AudioSpec mSampleFormat;
|
|
Uint8 * mSampleData;
|
|
Uint32 mSamplePos;
|
|
Uint32 mSampleLength;
|
|
bool playing;
|
|
|
|
public:
|
|
Sound(const std::string & path = "");
|
|
~Sound();
|
|
|
|
void init();
|
|
void deinit();
|
|
|
|
void loadFile(const std::string & path);
|
|
|
|
void play();
|
|
bool isPlaying() const;
|
|
void stop();
|
|
|
|
const Uint8 * getData() const;
|
|
Uint32 getPosition() const;
|
|
void setPosition(Uint32 newPosition);
|
|
Uint32 getLength() const;
|
|
};
|
|
|
|
#endif
|