mirror of
https://github.com/RetroDECK/ES-DE.git
synced 2025-01-21 00:05:37 +00:00
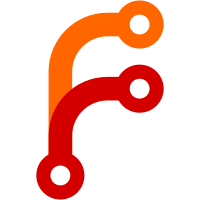
- Refactoring System Environment data - Added Virtual System Manager class - Added "all", "favorites" and "last played" systems - Added GuiInfoPopup class for notifications - Added Favorites to metadata, as well as a shortcut to toggle favorites - Added warning if enabling systems but themes don't support it - Added "filter by favorites" per system - Adjusted "Go to Random Game" behavior to account for the fact that we now have an "All Games" system - Added "sort by system name" for the collections
47 lines
1.5 KiB
C++
47 lines
1.5 KiB
C++
#pragma once
|
|
|
|
#include "FileData.h"
|
|
#include "Renderer.h"
|
|
|
|
class Window;
|
|
class GuiComponent;
|
|
class FileData;
|
|
class ThemeData;
|
|
|
|
// This is an interface that defines the minimum for a GameListView.
|
|
class IGameListView : public GuiComponent
|
|
{
|
|
public:
|
|
IGameListView(Window* window, FileData* root) : GuiComponent(window), mRoot(root)
|
|
{ setSize((float)Renderer::getScreenWidth(), (float)Renderer::getScreenHeight()); }
|
|
|
|
virtual ~IGameListView() {}
|
|
|
|
// Called when a new file is added, a file is removed, a file's metadata changes, or a file's children are sorted.
|
|
// NOTE: FILE_SORTED is only reported for the topmost FileData, where the sort started.
|
|
// Since sorts are recursive, that FileData's children probably changed too.
|
|
virtual void onFileChanged(FileData* file, FileChangeType change) = 0;
|
|
|
|
// Called whenever the theme changes.
|
|
virtual void onThemeChanged(const std::shared_ptr<ThemeData>& theme) = 0;
|
|
|
|
void setTheme(const std::shared_ptr<ThemeData>& theme);
|
|
inline const std::shared_ptr<ThemeData>& getTheme() const { return mTheme; }
|
|
|
|
virtual FileData* getCursor() = 0;
|
|
virtual void setCursor(FileData*) = 0;
|
|
|
|
virtual bool input(InputConfig* config, Input input) override;
|
|
virtual void remove(FileData* game, bool deleteFile) = 0;
|
|
|
|
virtual const char* getName() const = 0;
|
|
virtual void launch(FileData* game) = 0;
|
|
|
|
virtual HelpStyle getHelpStyle() override;
|
|
|
|
void render(const Eigen::Affine3f& parentTrans) override;
|
|
protected:
|
|
FileData* mRoot;
|
|
std::shared_ptr<ThemeData> mTheme;
|
|
};
|