mirror of
https://github.com/RetroDECK/ES-DE.git
synced 2024-11-24 07:05:39 +00:00
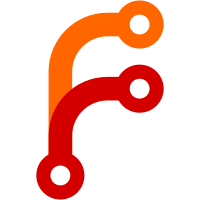
Fixed buttons not being quite vertically centered. Hooked up new switch graphics. Updated slider logic to only move in multiples of the specified increment.
42 lines
1 KiB
C++
42 lines
1 KiB
C++
#pragma once
|
|
|
|
#include "../GuiComponent.h"
|
|
#include "ImageComponent.h"
|
|
|
|
class TextCache;
|
|
class Font;
|
|
|
|
// Used to display/edit a value between some min and max values.
|
|
class SliderComponent : public GuiComponent
|
|
{
|
|
public:
|
|
//Minimum value (far left of the slider), maximum value (far right of the slider), increment size (how much just pressing L/R moves by), unit to display (optional).
|
|
SliderComponent(Window* window, float min, float max, float increment, const std::string& suffix = "");
|
|
|
|
void setValue(float val);
|
|
float getValue();
|
|
|
|
bool input(InputConfig* config, Input input) override;
|
|
void update(int deltaTime) override;
|
|
void render(const Eigen::Affine3f& parentTrans) override;
|
|
|
|
void onSizeChanged() override;
|
|
|
|
virtual std::vector<HelpPrompt> getHelpPrompts() override;
|
|
|
|
private:
|
|
void onValueChanged();
|
|
|
|
float mMin, mMax;
|
|
float mValue;
|
|
float mSingleIncrement;
|
|
float mMoveRate;
|
|
int mMoveAccumulator;
|
|
|
|
ImageComponent mKnob;
|
|
|
|
std::string mSuffix;
|
|
std::shared_ptr<Font> mFont;
|
|
std::shared_ptr<TextCache> mValueCache;
|
|
};
|