mirror of
https://github.com/RetroDECK/ES-DE.git
synced 2024-11-24 15:15:38 +00:00
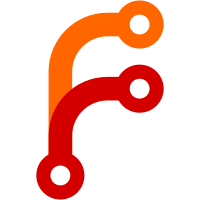
Scrolling will now occur if the input is held (not just keyboards anymore!). Initial XML gamelist support. If a file named gamelist.xml is present in the directory ES is run from, it will be parsed and the detailed GuiGameList will be used. Games are matched by absolute path, and a name, description, and image path can be read. PugiXML is used for parsing XML files - its license can be found in src/pugiXML/pugiXML_license.txt. SDL_image is used for loading screenshots with the detailed GuiGameList. Almost all invalid bash characters should be escaped in ROM paths now - including !$^&*()[]<>?;'"\.
54 lines
1.2 KiB
C++
54 lines
1.2 KiB
C++
#include "GameData.h"
|
|
#include <boost/filesystem.hpp>
|
|
#include <iostream>
|
|
|
|
bool GameData::isFolder() { return false; }
|
|
std::string GameData::getName() { return mName; }
|
|
std::string GameData::getPath() { return mPath; }
|
|
std::string GameData::getDescription() { return mDescription; }
|
|
std::string GameData::getImagePath() { return mImagePath; }
|
|
|
|
GameData::GameData(SystemData* system, std::string path, std::string name)
|
|
{
|
|
mSystem = system;
|
|
mPath = path;
|
|
mName = name;
|
|
|
|
mDescription = "";
|
|
mImagePath = "";
|
|
}
|
|
|
|
std::string GameData::getBashPath()
|
|
{
|
|
//a quick and dirty way to insert a backslash before most characters that would mess up a bash path
|
|
std::string path = mPath;
|
|
const char* invalidChars = " '\"\\!$^&*(){}[]?;<>";
|
|
for(unsigned int i = 0; i < path.length(); i++)
|
|
{
|
|
char c;
|
|
unsigned int charNum = 0;
|
|
do {
|
|
c = invalidChars[charNum];
|
|
if(path[i] == c)
|
|
{
|
|
path.insert(i, "\\");
|
|
i++;
|
|
break;
|
|
}
|
|
charNum++;
|
|
} while(c != '\0');
|
|
}
|
|
|
|
return path;
|
|
}
|
|
|
|
void GameData::set(std::string name, std::string description, std::string imagePath)
|
|
{
|
|
if(!name.empty())
|
|
mName = name;
|
|
if(!description.empty())
|
|
mDescription = description;
|
|
if(!imagePath.empty() && boost::filesystem::exists(imagePath))
|
|
mImagePath = imagePath;
|
|
}
|