mirror of
https://github.com/RetroDECK/ES-DE.git
synced 2025-01-30 12:05:39 +00:00
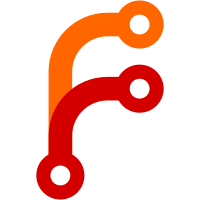
The ~/.emulationstation folder is now organized into categories. Everything probably broke again. Added support for "theme sets," instead of just one theme for each system. Read the top of THEMES.md for more information. Added support for reading from `/etc/emulationstation/` for themes, gamelists, and es_systems.cfg. Updated documentation to match.
79 lines
2.6 KiB
C++
79 lines
2.6 KiB
C++
#ifndef _SYSTEMDATA_H_
|
|
#define _SYSTEMDATA_H_
|
|
|
|
#include <vector>
|
|
#include <string>
|
|
#include "FileData.h"
|
|
#include "Window.h"
|
|
#include "MetaData.h"
|
|
#include "PlatformId.h"
|
|
#include "ThemeData.h"
|
|
|
|
class SystemData
|
|
{
|
|
public:
|
|
SystemData(const std::string& name, const std::string& fullName, const std::string& startPath, const std::vector<std::string>& extensions,
|
|
const std::string& command, PlatformIds::PlatformId platformId = PlatformIds::PLATFORM_UNKNOWN);
|
|
~SystemData();
|
|
|
|
inline FileData* getRootFolder() const { return mRootFolder; };
|
|
inline const std::string& getName() const { return mName; }
|
|
inline const std::string& getFullName() const { return mFullName; }
|
|
inline const std::string& getStartPath() const { return mStartPath; }
|
|
inline const std::vector<std::string>& getExtensions() const { return mSearchExtensions; }
|
|
inline PlatformIds::PlatformId getPlatformId() const { return mPlatformId; }
|
|
inline const std::shared_ptr<ThemeData>& getTheme() const { return mTheme; }
|
|
|
|
std::string getGamelistPath(bool forWrite) const;
|
|
bool hasGamelist() const;
|
|
std::string getThemePath() const;
|
|
|
|
unsigned int getGameCount() const;
|
|
|
|
void launchGame(Window* window, FileData* game);
|
|
|
|
static void deleteSystems();
|
|
static bool loadConfig(); //Load the system config file at getConfigPath(). Returns true if no errors were encountered. An example will be written if the file doesn't exist.
|
|
static void writeExampleConfig(const std::string& path);
|
|
static std::string getConfigPath(bool forWrite); // if forWrite, will only return ~/.emulationstation/es_systems.cfg, never /etc/emulationstation/es_systems.cfg
|
|
|
|
static std::vector<SystemData*> sSystemVector;
|
|
|
|
inline std::vector<SystemData*>::const_iterator getIterator() const { return std::find(sSystemVector.begin(), sSystemVector.end(), this); };
|
|
inline std::vector<SystemData*>::const_reverse_iterator getRevIterator() const { return std::find(sSystemVector.rbegin(), sSystemVector.rend(), this); };
|
|
|
|
inline SystemData* getNext() const
|
|
{
|
|
auto it = getIterator();
|
|
it++;
|
|
if(it == sSystemVector.end()) it = sSystemVector.begin();
|
|
return *it;
|
|
}
|
|
|
|
inline SystemData* getPrev() const
|
|
{
|
|
auto it = getRevIterator();
|
|
it++;
|
|
if(it == sSystemVector.rend()) it = sSystemVector.rbegin();
|
|
return *it;
|
|
}
|
|
|
|
// Load or re-load theme.
|
|
void loadTheme();
|
|
|
|
private:
|
|
std::string mName;
|
|
std::string mFullName;
|
|
std::string mStartPath;
|
|
std::vector<std::string> mSearchExtensions;
|
|
std::string mLaunchCommand;
|
|
PlatformIds::PlatformId mPlatformId;
|
|
std::shared_ptr<ThemeData> mTheme;
|
|
|
|
void populateFolder(FileData* folder);
|
|
|
|
FileData* mRootFolder;
|
|
};
|
|
|
|
#endif
|