mirror of
https://github.com/RetroDECK/Supermodel.git
synced 2025-03-06 14:27:44 +00:00
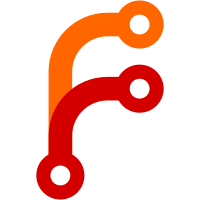
- Fixed bug with mapping of multiple assignments. - Added new ! operator for input mappings, which lets the user specify that an input must not be active. - Added option to print info about input system (such as settings and detected keyboards, mice and joysticks) during input configuration. - Added new trigger input for lightgun games with a configurable option to automatically pull trigger when offscreen input is activated (this makes playing with the mouse easier as the gun can be reloaded with single mouse button, rather than having to press both buttons at the same time). - Added -xinput command line option that switches to using XInput API rather than DirectInput for XBox 360 controllers (this allows the XBox 360 controller's two triggers to be read independently which works better for driving games when they are mapped to accele rator and brake). - Added initial version of force feedback implementation to DirectInputSystem (this still needs work).
114 lines
2.1 KiB
C++
114 lines
2.1 KiB
C++
#ifndef INCLUDED_SDLINPUTSYSTEM_H
|
|
#define INCLUDED_SDLINPUTSYSTEM_H
|
|
|
|
#include "Types.h"
|
|
#include "Inputs/InputSource.h"
|
|
#include "Inputs/InputSystem.h"
|
|
|
|
#ifdef SUPERMODEL_OSX
|
|
#include <SDL/SDL.h>
|
|
#else
|
|
#include <SDL.h>
|
|
#endif
|
|
|
|
#include <vector>
|
|
using namespace std;
|
|
|
|
#define NUM_SDL_KEYS (sizeof(s_keyMap) / sizeof(SDLKeyMapStruct))
|
|
|
|
struct SDLKeyMapStruct
|
|
{
|
|
const char *keyName;
|
|
SDLKey sdlKey;
|
|
};
|
|
|
|
/*
|
|
* Input system that uses SDL.
|
|
*/
|
|
class CSDLInputSystem : public CInputSystem
|
|
{
|
|
private:
|
|
// Lookup table to map key names to SDLKeys
|
|
static SDLKeyMapStruct s_keyMap[];
|
|
|
|
// Vector to keep track of attached joysticks
|
|
vector<SDL_Joystick*> m_joysticks;
|
|
|
|
// Vector of joystick details
|
|
vector<JoyDetails> m_joyDetails;
|
|
|
|
// Current key state obtained from SDL
|
|
Uint8 *m_keyState;
|
|
|
|
// Current mouse state obtained from SDL
|
|
int m_mouseX;
|
|
int m_mouseY;
|
|
int m_mouseZ;
|
|
short m_mouseWheelDir;
|
|
Uint8 m_mouseButtons;
|
|
|
|
/*
|
|
* Opens all attached joysticks.
|
|
*/
|
|
void OpenJoysticks();
|
|
|
|
/*
|
|
* Closes all attached joysticks.
|
|
*/
|
|
void CloseJoysticks();
|
|
|
|
protected:
|
|
/*
|
|
* Initializes the SDL input system.
|
|
*/
|
|
bool InitializeSystem();
|
|
|
|
int GetKeyIndex(const char *keyName);
|
|
|
|
const char *GetKeyName(int keyIndex);
|
|
|
|
bool IsKeyPressed(int kbdNum, int keyIndex);
|
|
|
|
int GetMouseAxisValue(int mseNum, int axisNum);
|
|
|
|
int GetMouseWheelDir(int mseNum);
|
|
|
|
bool IsMouseButPressed(int mseNum, int butNum);
|
|
|
|
int GetJoyAxisValue(int joyNum, int axisNum);
|
|
|
|
bool IsJoyPOVInDir(int joyNum, int povNum, int povDir);
|
|
|
|
bool IsJoyButPressed(int joyNum, int butNum);
|
|
|
|
bool ProcessForceFeedbackCmd(int joyNum, int axisNum, ForceFeedbackCmd *ffCmd);
|
|
|
|
void Wait(int ms);
|
|
|
|
public:
|
|
/*
|
|
* Constructs an SDL input system.
|
|
*/
|
|
CSDLInputSystem();
|
|
|
|
~CSDLInputSystem();
|
|
|
|
int GetNumKeyboards();
|
|
|
|
int GetNumMice();
|
|
|
|
int GetNumJoysticks();
|
|
|
|
const KeyDetails *GetKeyDetails(int kbdNum);
|
|
|
|
const MouseDetails *GetMouseDetails(int mseNum);
|
|
|
|
const JoyDetails *GetJoyDetails(int joyNum);
|
|
|
|
bool Poll();
|
|
|
|
void SetMouseVisibility(bool visible);
|
|
};
|
|
|
|
#endif // INCLUDED_SDLINPUTSYSTEM_H
|