mirror of
https://github.com/RetroDECK/Supermodel.git
synced 2024-11-24 06:35:41 +00:00
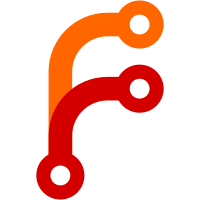
The old texture code was being bottle necked by the texture reads. We mirrored the real3d texture memory directly, including the mipmaps in a single large texture. I *think* most h/w has some sort of texture cache for a 2x2 or 4x4 block of pixels for a texture. What we were doing was reading the base texture, then reading the mipmap data from a totally separate part of the same texture which I can only assume flushed this cache. What I did was to create mipmap chains for the texture sheet, then copy the mipmap data there. Doing this basically doubles performance.
34 lines
579 B
C++
34 lines
579 B
C++
#pragma once
|
|
|
|
#ifndef _TEXTUREBANK_H_
|
|
#define _TEXTUREBANK_H_
|
|
|
|
#include "Types.h"
|
|
#include <GL/glew.h>
|
|
|
|
// texture banks are a fixed size
|
|
// 2048x1024 pixels, each pixel is 16bits in size
|
|
|
|
namespace New3D {
|
|
|
|
class TextureBank
|
|
{
|
|
public:
|
|
TextureBank();
|
|
~TextureBank();
|
|
|
|
void AttachMemory(const UINT16* textureRam);
|
|
void Bind();
|
|
void UploadTextures(int level, int x, int y, int width, int height);
|
|
int GetNumberOfLevels();
|
|
|
|
private:
|
|
GLuint m_texID = 0;
|
|
const UINT16* m_textureRam = nullptr;
|
|
int m_numLevels = 0;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|