mirror of
https://github.com/RetroDECK/Supermodel.git
synced 2024-11-22 22:05:38 +00:00
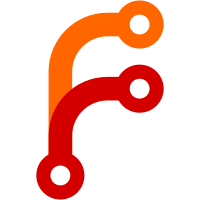
Late christmas present. Due to the way alpha works on the model3 adding regular anti-aliasing doesn't really work. Supersampling is very much a brute force solution, render the scene at a higher resolution and mipmap it. It's enabled via command line with the -ss option, for example -ss=4 for 4x supersampling or by adding Supersampling = 4 in the config file. Note non power of two values work as well, so 3 gives a very good balance between speed and quality. 8 will make your GPU bleed, since it is essentially rendering 64 pixels for every visible pixel on the screen.
31 lines
1,016 B
C++
31 lines
1,016 B
C++
#ifndef INCLUDED_IRENDER3D_H
|
|
#define INCLUDED_IRENDER3D_H
|
|
|
|
#include <cstdint>
|
|
|
|
/*
|
|
* IRender3D:
|
|
*
|
|
* Interface (abstract base class) for Real3D rendering engine.
|
|
*/
|
|
class IRender3D
|
|
{
|
|
public:
|
|
virtual void RenderFrame(void) = 0;
|
|
virtual void BeginFrame(void) = 0;
|
|
virtual void EndFrame(void) = 0;
|
|
virtual void UploadTextures(unsigned level, unsigned x, unsigned y, unsigned width, unsigned height) = 0;
|
|
virtual void AttachMemory(const uint32_t *cullingRAMLoPtr, const uint32_t *cullingRAMHiPtr, const uint32_t *polyRAMPtr, const uint32_t *vromPtr, const uint16_t *textureRAMPtr) = 0;
|
|
virtual void SetStepping(int stepping) = 0;
|
|
virtual bool Init(unsigned xOffset, unsigned yOffset, unsigned xRes, unsigned yRes, unsigned totalXRes, unsigned totalYRes, unsigned aaTarget) = 0;
|
|
virtual void SetSunClamp(bool enable) = 0;
|
|
virtual void SetSignedShade(bool enable) = 0;
|
|
virtual float GetLosValue(int layer) = 0;
|
|
|
|
virtual ~IRender3D()
|
|
{
|
|
}
|
|
};
|
|
|
|
#endif // INCLUDED_IRENDER3D_H
|